For example, a computer program has a character stored from a-z. It gives to user five chances or tries to guess the character. In this case, the task of guessing the character must be performed at least once. To ensure that a block of statements is executed at least once, C provides a do-while structure. The syntax of do-while structure is as under:
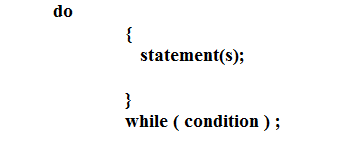
Here we see that the condition is tested after executing the statements of the loop body. Thus, the loop body is executed at least once and then the condition in do while statement is tested. If it is true, the execution of the loop body is repeated. In case, it proves otherwise (i.e. false), then the control goes to the statement next to the do while statement. This structure describes ‘execute the statements enclosed in braces in do clause when the condition in while clause is true.
Broadly speaking, in while loop, the condition is tested at the beginning of the loop before the body of the loop is performed. Whereas in do-while loop, the condition is tested after the loop body is performed. Therefore, in do-while loop, the body of the loop is executed at least once.
The flow chart of do-while structure is as follow:
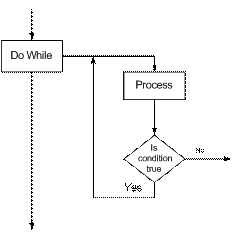
Example
Let’s consider the example of guessing a character. We have a character in the program to be guessed by the user. Let’s call it ‘z’. The program allows five tries (chances) to the user to guess the character. We declare a variable tryNum to store the number of tries. The program prompts the user to enter a character for guessing. We store this character in a variable c.
We declare the variable c of type char. The data type char is used to store a single character. We assign a character to a variable of char type by putting the character in single quotes. Thus the assignment statement to assign a value to a char variable will be as c = ‘a’. Note that there should be a single character in single quotes. The statement like c = ‘gh’ will be a syntax error.
Here we use the do-while construct. In the do clause we prompt the user to enter a character. After getting character in variable c from user, we compare it with our character i.e ‘z’. We use if\else structure for this comparison. If the character is the same as ours then we display a message to congratulate the user else we add 1 to tryNum variable. And then in while clause, we test the condition whether tryNum is less than or equal to 5 (tryNum <= 5). If this condition is true, then the body of the do clause is repeated again. We do this only when the condition (tryNum <= 5) remains true. If it is otherwise, the control goes to the first statement after the do-while loop. If guess is matched in first or second try, then we should exit the loop. We know that the loop is terminated when the condition tryNum <= 5 becomes false, so we assign a value which is greater than 5 to tryNum after displaying the message. Now the condition in the while statement is checked. It proves false (as tryNum is greater than 5). So the control goes out of the loop. First look here the flow chart for the program.
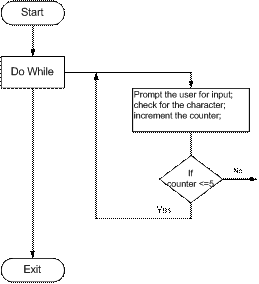
The code of the program is given below.
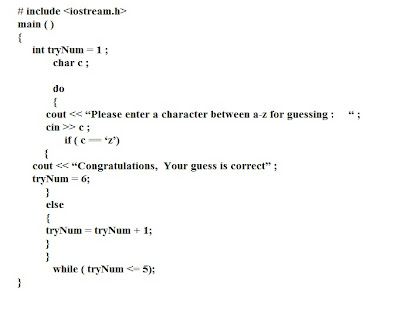
There is an elegant way to exit the loop when the correct number is guessed. We change the condition in while statement to a compound condition. This condition will check whether the number of tries is less than or equal to 5 and the variable c is not equal to ‘z’. So we will write the while clause as while (tryNum <= 5 && c != ‘z’ ); Thus when a single condition in this compound condition becomes false, then the control will exit the loop. Thus we need not to assign a value greater than 5 to variable tryNum. Thus the code of the program will be as:
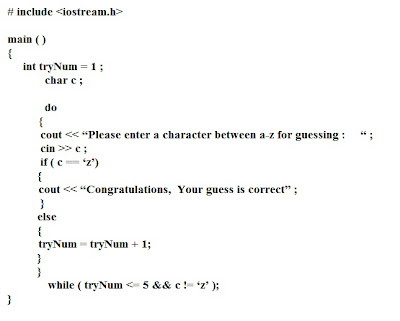
The output of the program is given below.

Here is another out put of the same program
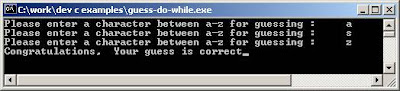
For previous lesson click here: while exercises
For next lesson click here: coming soon
the easiest way to learn programming
introduction to programming
do-while Statement
0 comments:
Post a Comment